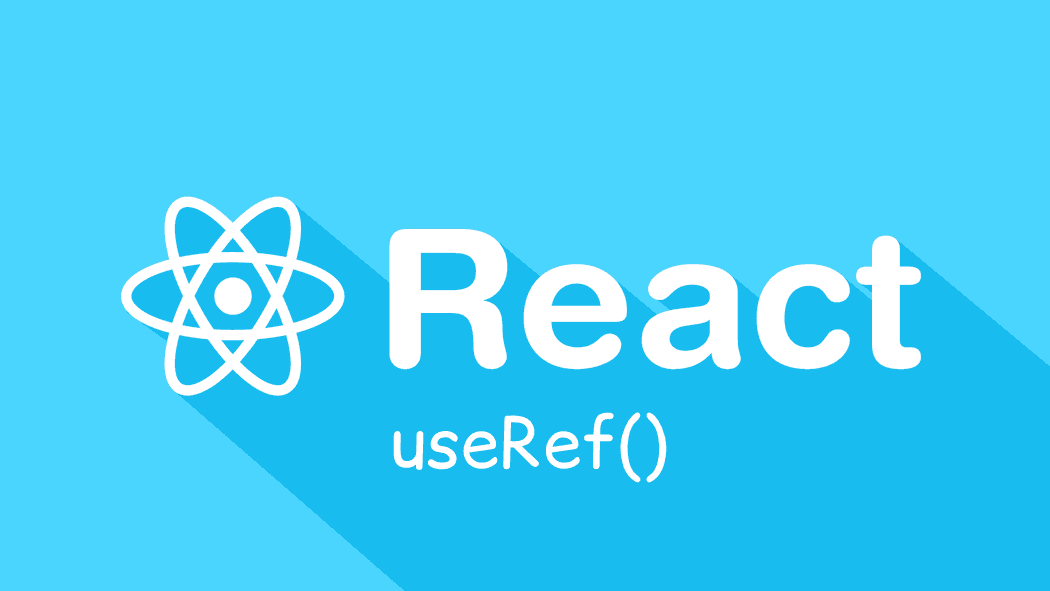
1. Understanding useRef in React
The useRef hook in React is often overlooked but can be incredibly powerful when used effectively. While it is commonly used for accessing DOM elements, there are several advanced use cases that many developers might not know. This article will dive into these use cases and explain why useRef is an essential tool for React developers.
1.1 What is useRef?
In simple terms, useRef is a hook that provides a way to persist values across renders without causing re-renders when the value changes. It returns an object with a current property, which you can update directly.
Think of useRef as a way to create a mutable container that survives the component's lifecycle.
1.2 Basic Usage
The most common use of useRef is to interact with the DOM. For example:
You can access and manipulate a DOM element without triggering a re-render.
Ensure you only use useRef for direct DOM manipulation when absolutely necessary, as it can bypass React's declarative nature.
import React, { useRef } from 'react';
function FocusInput() {
const inputRef = useRef(null);
const handleFocus = () => {
inputRef.current.focus();
};
return (
<div>
<input ref={inputRef} type="text" />
<button onClick={handleFocus}>Focus Input</button>
</div>
);
}
2. Advanced Use Cases
While the basic use case is well-known, here are some advanced scenarios where useRef shines.
2.1 Storing Previous Values
useRef can store the previous state or props value for comparison in functional components. This is useful when you need to detect changes.
import React, { useRef, useEffect } from 'react';
function PreviousValueTracker({ value }) {
const prevValue = useRef();
useEffect(() => {
prevValue.current = value;
}, [value]);
return (
<div>
<p>Current Value: {value}</p>
<p>Previous Value: {prevValue.current}</p>
</div>
);
}
2.2 Managing Intervals or Timeouts
useRef is perfect for managing intervals or timeouts without causing re-renders.
import React, { useRef, useEffect } from 'react';
function Timer() {
const intervalRef = useRef();
useEffect(() => {
intervalRef.current = setInterval(() => {
console.log('Tick');
}, 1000);
return () => clearInterval(intervalRef.current);
}, []);
return <div>Check the console for ticks!</div>;
}
2.3 Avoiding Re-Renders with Mutable Variables
Sometimes, you need to store mutable variables that don't trigger re-renders. This is where useRef is extremely useful.
import React, { useState, useRef } from 'react';
function Counter() {
const count = useRef(0);
const [trigger, setTrigger] = useState(false);
const increment = () => {
count.current += 1;
console.log('Count:', count.current);
};
return (
<div>
<button onClick={increment}>Increment</button>
<button onClick={() => setTrigger(!trigger)}>Trigger Render</button>
</div>
);
}
2.4 Creating Custom Hooks
useRef is a great tool for building custom hooks. For instance, you can track the mounted state of a component to prevent memory leaks.
import { useRef, useEffect } from 'react';
function useIsMounted() {
const isMounted = useRef(true);
useEffect(() => {
return () => {
isMounted.current = false;
};
}, []);
return isMounted;
}
3. Conclusion
The useRef hook is far more versatile than most developers realize. From managing DOM interactions to tracking previous values and managing intervals, it’s a powerful tool in the React ecosystem. By understanding and leveraging these advanced use cases, you can unlock its full potential and write more efficient, clean, and effective React components.